Basic Data Structures
My Take on UCSD's Basic Data Structures course (CSE 12)
Project maintained by nate-browne
Hosted on GitHub Pages — Theme by mattgraham
Style Guide for CSE 12
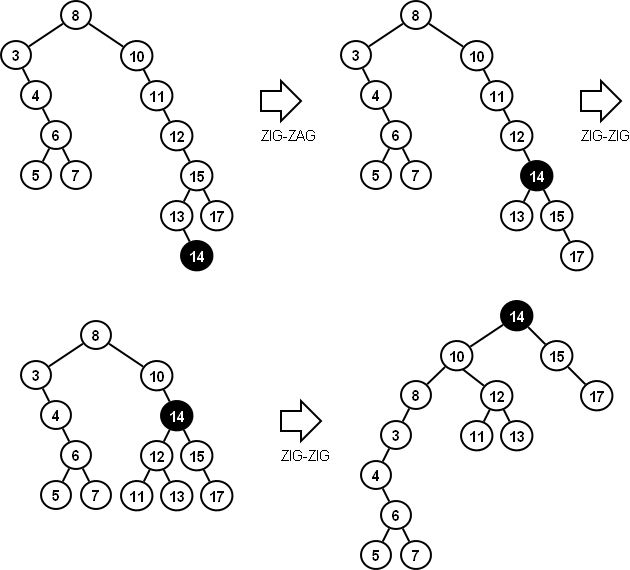
The style guide for this class is largely based on the Google C++ Style Guide,
with a few modifications/simplifications. In general though, you can just follow what it says to do.
- In general, you should include C library header files first, then C++ library headers, other library header files
(not relevant for this class), and lastly the header files for this project.
- You can
inline
getter and setter functions, though you don’t have to.
- Don’t use external libraries unless told to (so no usage of
boost
).
Classes
- Structs vs Classes
- Use structs only for passive objects that carry data, but use classes for everything else.
- For functors, you can use structs (probably won’t be relevant for this course).
- Inheritance
- We’ll prefer composition over inheritance, but only do
public
derivations if inheritance is being used.
- If you need a
private
derivation, just use composition.
- Operator Overloading
- Use it. It’s good.
- For classes we write, we’ll tell you which operators to overload.
public
, private
, protected
- The code should be two spaces, but the keyword itself should be one space indented.
use this link as an example.
- Tabs vs Spaces
- Use spaces. 2 or 4 spaces is fine (2 preferred) but absolutely no tabs.
- Set up your editor to replace tabs with spaces.
- Line Length
- Traditionally, line lengths were capped at 80. This is mostly due to legacy
reasons, and as such we will not be enforcing this rule in this course.
- Function Declarations and Definitions
- Put them all on the same line, if they fit.
- If it doesn’t fit, refer to this link.
- Function Calls
- Conditionals
- Refer to this link, except that it’s fine to do
if(condition)
without the space after the if
.
- Loops and Switch Statements
- This link will be your friend here. Just don’t put blank bodies of code.
- Pointer and References
- Don’t put spaces around the period or arrow, and don’t put trailing spaces after the pointer.
Naming
- Constants/Macros
- These should be UPPER_SNAKE_CASE.
- Variable Names
- Like file names, these should be snake_case. Don’t use lowerCamelCase
- Namespaces
- These should be all lowercase (e.g.
std::
and test_utils
)
- File Headers
- Every file needs a header containing name, account number, date, assignment number, sources of help, and a short description. The formatting can be however you want, though.
- Class Headers
- Every class needs a header explaining the usage, methods, and purpose. Formatting can be however you want as long as all of the information is present and it doesn’t look bad.
- Commenting in Methods
- Avoid overcommenting. Make sure you comment big ideas as well as particularly confusing bits of your code, but assume your reader understands advanced C++ (cause they do) so you don’t need to comment bits like
x++
.